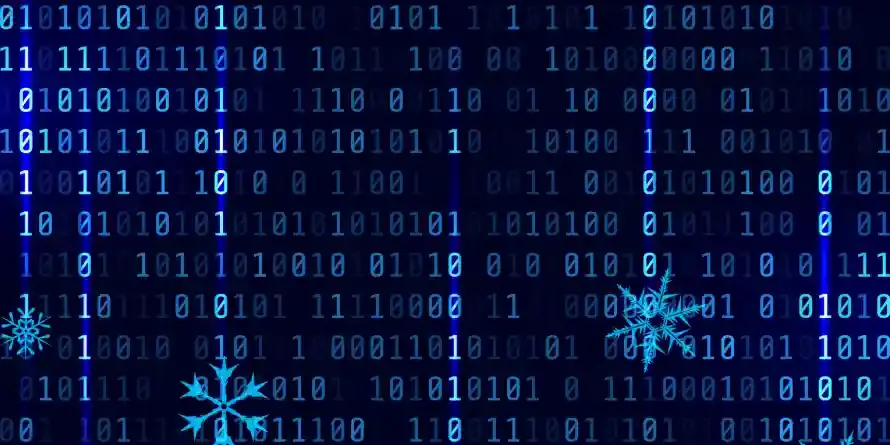
Git Bash Commands
Before you dive in
Notes:
-- Exclude block brackets in commands
-- Directory => folder
-- Remote repository => GitHub repository
-- <git init> is to be run in a folder you created
cd [Documents] => to change directory e.g. from root to Documents ls => list items in directory
mkdir [randomfolder] => to create a folder with name randomfolder
git init => run in desired folder (e.g. randomfolder) to make folder a Git local repository
touch [file name] => to create a file (include file extension, e.g. file.txt)
git rm -rf [folder name] => to delete a folder
git rm [file name] => to delete a file (include file extension, e.g. file.txt)
git status => to check status e.g. to commits made
touch .gitignore => create this (folder) to store static (large) files
git rm -r --cached . => to remove files in .gitignore folder from being remembered/cache
git add . => add all files in directory to staging area
git add [filename] => to add a file to staging area
git add -A => to add new and updated files to staging area
git restore --staged [file name] => to unstage file
git rm -f [file name] => force remove file from working directory
git rm --cached [file name] => remove file from staging area
Before you start committing, you may need to do some configurations like those below:
git config --global user.email "youremail@email.com" and
git config --global user.name "youremail"
Then:
git commit -m "[commit message. e.g. "Initial commit"]" => to commit changes (include quotes)
git commit -a -m "[commit message. e.g. "Commit tracked files"]" => to commit tracked files only
git log => to view history/changes
git checkout [commit id] => to switch to desired commit
git branch [branch name] => to create a new branch
git branch -d [branch name] => to delete a branch
git branch => to view list of branches (the one with asterisk is the current branch)
git branch -a => check lists of branches
git checkout [branch name] => to switch to a branch
git checkout master => switch to master branch
git checkout -b [new-branch-name] => to create a new branch and switch to it
git checkout - => to switch to branch last checked out
git merge [branch name] => to merge branch into active branch
git merge [branch name] [target branch] => to merge a branch into a target branch
git revert [commit id] => goes back one commit and creates another commit
git checkout [commit id] => to reverse revert
git remote add origin [https link from GitHub repo] => to add a remote repository
git remote -v => should show GitHub repository's fetch and push which means there is connection
git pull => to update local repository with changes in remote repository
git pull origin [branch name] => to fetch/pull from a remote repository's branch to your local
git pull origin master => to fetch/pull from a remote repository's master branch to your local
git push origin [branch name] => push a branch to a remote repository
git push -u origin [branch name] => push to remote repository's branch (and remember the branch)
git push => push to remembered remote repository